Python - Tuples
A Tuple is a type of data container in Python which is used to store multiple data in one variable. It can contain elements of different data types. Elements in a tuple are ordered and can be accessed using it's index number. Unlike lists, tuples are immutable and hence elements of a tuple are not changeable.
Create Tuple
Tuple can be created by separating it's elements by comma , and enclosing with round brackets ( ). Additionally, it can also be created using tuple() function.
#Tuple with multiple datatypes Info = ('John', 25, 'London') print(Info) #Creating tuple using constructor colors = tuple(('Red', 'Blue', 'Green')) print(colors)
The output of the above code will be:
('John', 25, 'London') ('Red', 'Blue', 'Green')
Access element of a Tuple
An element of a tuple can be accessed with it's index number. Index number for tuple in Python starts with 0 in forward direction and -1 in backward direction. The figure below describes the indexing concept of a tuple.
Tuple Indexing:
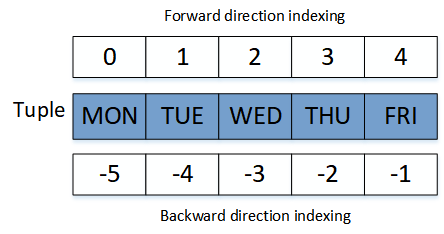
The example below describes how to access elements of a tuple using its index number.
weekday = ('MON', 'TUE', 'WED', 'THU', 'FRI') #forward indexing print(weekday[1]) #backward indexing print(weekday[-1])
The output of the above code will be:
TUE FRI
Access range of elements of a Tuple
Range of elements of a tuple can be selected using statement like [startIndex : endIndex] where end_index is excluded. If start_index or end_index are not mentioned then it takes first and last index numbers of the tuple respectively.
weekday = ('MON', 'TUE', 'WED', 'THU', 'FRI') print(weekday[1:3]) print(weekday[-5:-1],"\n") print(weekday[1:]) print(weekday[:-3],"\n") print(weekday[:])
The output of the above code will be:
('TUE', 'WED') ('MON', 'TUE', 'WED', 'THU') ('TUE', 'WED', 'THU', 'FRI') ('MON', 'TUE') ('MON', 'TUE', 'WED', 'THU', 'FRI')
Modify value of an Element
Tuple's elements are immutable and unchangeable. However, there is a way around to achieve this. First, change tuple into list using list() function, make required changes and finally, convert it back to tuple using tuple() function.
Info = ('John', 25, 'London') #tuple converted into list Info = list(Info) #Making required changes Info[0] = 'Marry' #list converted back to tuple Info = tuple(Info) print(Info)
The above code will give the following output:
('Marry', 25, 'London')
Add / Delete elements of a Tuple
Tuple's elements are immutable and unchangeable. Therefore it is not possible to delete or modify elements after creating the tuple.
month = ('JAN', 'FEB', 'MAR') # returns an error month[3] = 'APR' print(month)
The output of the above code will be:
TypeError: 'tuple' object does not support item assignment
Similarly, it is not possible to delete an element of a tuple.
month = ('JAN', 'FEB', 'MAR') # returns an error del month[2] print(month)
The output of the above code will be:
Traceback (most recent call last): File "Main.py", line 3, in <module> del month[2] TypeError: 'tuple' object doesn't support item deletion
However, the tuple can be deleted itself using del keyword.
month = ('JAN', 'FEB', 'MAR') # delete tuple completely del month print(month)
The output of the above code will be:
Traceback (most recent call last): File "Main.py", line 4, in <module> print(month) NameError: name 'month' is not defined
Tuple Length
The len() function can be used to find out total number of elements in a list, tuple, set or dictionary.
number = (10, 50, 50, 100, 1000, 1000) print(len(number))
The output of the above code will be:
6
Loop over Tuple
For loop over Tuple:
for loop can be used to access each element of a tuple.
colors = ('Red', 'Blue', 'Green') for x in colors: print(x)
The output of the above code will be:
Red Blue Green
While loop over Tuple
By using while loop and len() function, each element of a tuple can be accessed.
colors = ('Red', 'Blue', 'Green') i = 0 while i < len(colors): print(colors[i]) i = i + 1
The above code will give the following output:
Red Blue Green
Check an element in the Tuple
colors = ('Red', 'Blue', 'Green') if 'white' in colors: print('Yes, white is an element of colors.') else: print('No, white is not an element of colors.')
The above code will give the following output:
No, white is not an element of colors.
Join Tuples
The + operator can be used to join two tuples into a new tuple.
colors = ('Red', 'Blue', 'Green') numbers = (10, 20) mytuple = colors + numbers print(mytuple)
The output of the above code will be:
('Red', 'Blue', 'Green', 10, 20)
Single Element Tuple
Add comma , after the element to create single element tuple.
#this is tuple color = ('Red',) print(type(color)) #this is string color = ('Red') print(type(color))
The output of the above code will be:
<class 'tuple'> <class 'str'>