Python - Arrays
Note: Python does not have inbuilt array support, but the Python Lists can be used as an array.
The Array is a type of data container which is used to store multiple data elements in one variable. Elements of the array can be accessed using its index number.
Create an Array
Array (List) can be created by separating it's elements using comma , and enclosing them with square bracket [ ].
Info = ['John', 'Marry', 'Kim'] print(Info)
The output of the above code will be:
['John', 'Marry', 'Kim']
Access element of an Array
An element of an array can be accessed with it's index number. In Python, index number starts with 0 in forward direction and -1 in backward direction. The figure below describes the indexing concept of an array.
Array Indexing:
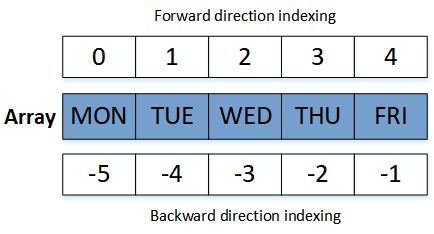
The example below describes how to access elements of an array using its index number.
weekday = ['MON', 'TUE', 'WED', 'THU', 'FRI'] #forward indexing print(weekday[1]) #backward indexing print(weekday[-1])
The output of the above code will be:
TUE FRI
Access range of elements of an Array
Range of elements of an array can be selected using statement like [startIndex : endIndex] where end_index is excluded. If start_index and end_index are not mentioned then it takes first and last index numbers of the array respectively.
weekday = ['MON', 'TUE', 'WED', 'THU', 'FRI'] print(weekday[1:3]) print(weekday[-5:-1],"\n") print(weekday[1:]) print(weekday[:-3],"\n") print(weekday[:])
The output of the above code will be:
['TUE', 'WED'] ['MON', 'TUE', 'WED', 'THU'] ['TUE', 'WED', 'THU', 'FRI'] ['MON', 'TUE'] ['MON', 'TUE', 'WED', 'THU', 'FRI']
Modify value of an Element
To change element's value, assign new value using it's index.
Info = ['John', 'Marry', 'Kim'] #value at index=0 changed to 'Jo' Info[0] = 'Jo' print(Info)
The above code will give the following output:
['Jo', 'Marry', 'Kim']
Add elements in an Array
Below mentioned methods are used to add elements in an array:
- append() - add an element to the end of an array.
- insert() - insert an element at specified index of the array.
days = ['MON', 'TUE', 'WED', 'THU', 'FRI', 'SAT'] # add this element in last of the array days.append('SUN') print(days) month = ['JAN', 'FEB', 'MAR', 'MAY'] # add 'APR' at index=3 of the array month.insert(3,'APR') print(month)
The output of the above code will be:
['MON', 'TUE', 'WED', 'THU', 'FRI', 'SAT', SUN'] ['JAN', 'FEB', 'MAR', 'APR', 'MAY']
Delete elements of an Array
Below mentioned methods/keywords is used to delete elements from an array:
- remove() - deletes first occurrence of a specified element from the array.
- pop() - deletes element at specified index or last element if index is not specified.
- clear() - deletes all elements of an array.
- del - deletes a element or range of elements or array itself.
number = [10, 50, 50, 100, 1000] #delete first occurrence of 50. number.remove(50) print(number) number = [10, 50, 50, 100, 1000] #delete element at index=3. number.pop(3) print(number) number = [10, 50, 50, 100, 1000] #delete last element from the array. number.pop() print(number) number = [10, 50, 50, 100, 1000] #delete all elements from the array. number.clear() print(number)
The above code will give the following output:
[10, 50, 100, 1000] [10, 50, 50, 1000] [10, 50, 50, 100] []
The del keyword is used to delete an element or range of elements or array itself.
number = [10, 50, 50, 100, 1000] #delete element at index=3. del number[3] print(number) number = [10, 50, 50, 100, 1000] #delete element at index=1,2 and 3. del number[1:4] print(number) number = [10, 50, 50, 100, 1000] #delete array 'number'. del number print(number)
The above code will give the following output:
[10, 50, 50, 1000] [10, 1000] Traceback (most recent call last): File "Main.py", line 14, in <module> print(number) NameError: name 'number' is not defined
Array length
The len() function can be used to find out total number of elements in an array.
number = [10, 50, 50, 100, 1000, 1000] print(len(number))
The output of the above code will be:
6
Loop over Array
For loop over array:
In the example below, for loop is used to access all elements of the given array.
colors = ['Red', 'Blue', 'Green'] for x in colors: print(x)
The output of the above code will be:
Red Blue Green
While loop over Array
By using while loop and len() function, each element of an array can be accessed.
colors = ['Red', 'Blue', 'Green'] i = 0 while i < len(colors): print(colors[i]) i = i + 1
The above code will give the following output:
Red Blue Green
Check an element in the Array
If control statement is used to check whether the array contains specified element or not.
colors = ['Red', 'Blue', 'Green'] if 'white' in colors: print('Yes, white is an element of colors.') else: print('No, white is not an element of colors.')
The above code will give the following output:
No, white is not an element of colors.