Python - For Loop
The for loop
The for loop in Python is used to iterate over a given sequence and executes a set of statements for each element in the sequence. A sequence can be any data structure like list, tuple, set, string, dictionary and range iterables etc.
Syntax
for iterating_var in sequence: statements
Flow Diagram:
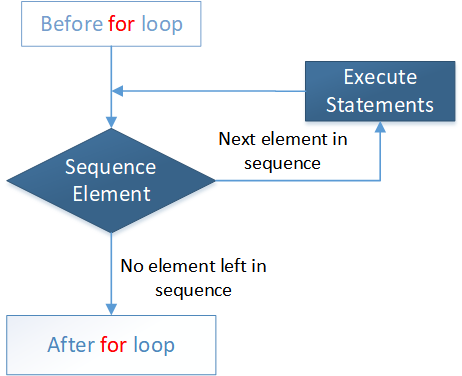
For loop over a range:
In the example below, for loop is used over a given range.
print("#Result 1:") for i in range(4): print(i, i * 2) print("\n#Result 2:") for j in range(2, 6): print(j, j ** 2) print("\n#Result 3:") for k in range(10, 2, -2): print(k)
The output of the above code will be:
#Result 1: 0 0 1 2 2 4 3 6 #Result 2: 2 4 3 9 4 16 5 25 #Result 3: 10 8 6 4
For loop over List
In the example below, for loop is used over a list to access its elements.
colors = ['Red', 'Blue', 'Green'] for x in colors: print(x)
The output of the above code will be:
Red Blue Green
For loop over Tuple
In the example below, for loop is used over a tuple to access its elements.
days = ('Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday', 'Sunday') for day in days: print(day)
The output of the above code will be:
Monday Tuesday Wednesday Thursday Friday Saturday Sunday
For loop over Set
The example below describes how to use a for loop over a set to access its elements.
numbers = {100, 200, 300, 400, 500} for i in numbers: print(i)
The output of the above code will be:
400 100 500 200 300
For loop over String
As discussed earlier, a for loop can be used to access characters of a given string.
word = 'python' for letter in word: print(letter)
The output of the above code will be:
p y t h o n
For loop over Dictionary
In the same way, a for loop can be used to access key-value pairs of a given dictionary.
dict = { 'year': '2000', 'month': 'March', 'date': 15 } print("#Result 1:") for day in dict: print(day) print("\n#Result 2:") for day, value in dict.items(): print(day,value)
The output of the above code will be:
#Result 1: year month date #Result 2: year 2000 month March date 15