SciPy - Exponential Distribution
Exponential distribution is the probability distribution of the time between events in a Poisson point process, i.e., a process in which events occur continuously and independently at a constant average rate. It is a particular case of the gamma distribution. For example, customers arriving at a store, file requests on a server etc.
The probability density function (pdf) of exponential distribution is defined as:
Where, β is the scale parameter which is the inverse of the rate parameter λ = 1/β.
An exponential distribution has mean β and variance β2.
The cumulative distribution function (cdf) evaluated at x, is the probability that the random variable (X) will take a value less than or equal to x. The cdf of exponential distribution is defined as:
The scipy.stats.expon contains all the methods required to generate and work with an exponential distribution. The most frequently methods are mentioned below:
Syntax
scipy.stats.expon.pdf(x, loc=0, scale=1) scipy.stats.expon.cdf(x, loc=0, scale=1) scipy.stats.expon.ppf(q, loc=0, scale=1) scipy.stats.expon.rvs(loc=0, scale=1, size=1)
Parameters
x |
Required. Specify float or array_like of floats representing random variable. |
q |
Required. Specify float or array_like of floats representing probabilities. |
loc |
Optional. Specify the location of the distribution. Default is 0. |
scale |
Optional. Specify the scale parameter, β = 1/λ. Must be non-negative. Default is 1.0. |
size |
Optional. Specify output shape. |
expon.pdf()
The expon.pdf() function measures probability density function (pdf) of the distribution.
from scipy.stats import expon import matplotlib.pyplot as plt import numpy as np #creating an array of values between #-1 to 10 with a difference of 0.1 x = np.arange(-1, 10, 0.1) y = expon.pdf(x, 0, 2) plt.plot(x, y) plt.show()
The output of the above code will be:
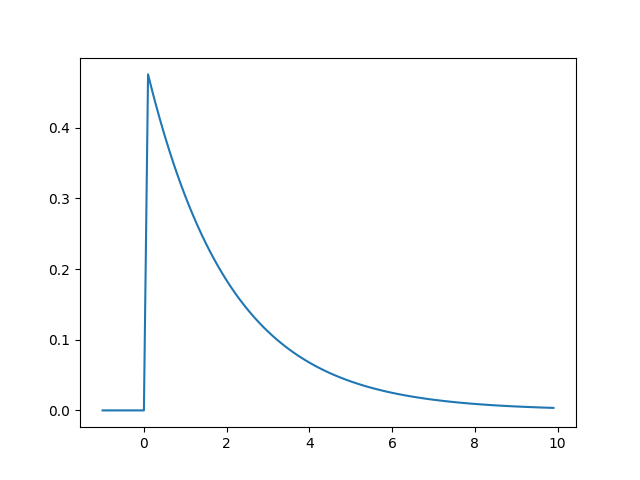
expon.cdf()
The expon.cdf() function returns cumulative distribution function (cdf) of the distribution.
from scipy.stats import expon import matplotlib.pyplot as plt import numpy as np #creating an array of values between #-1 to 10 with a difference of 0.1 x = np.arange(-1, 10, 0.1) y = expon.cdf(x, 0, 2) plt.plot(x, y) plt.show()
The output of the above code will be:
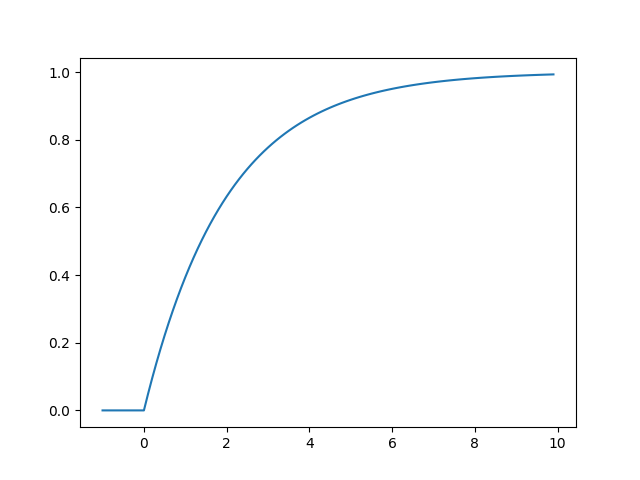
expon.ppf()
The expon.ppf() function takes the probability value and returns cumulative value corresponding to probability value of the distribution.
from scipy.stats import expon import matplotlib.pyplot as plt import numpy as np #creating an array of probability from #0 to 1 with a difference of 0.01 x = np.arange(0, 1, 0.01) y = expon.ppf(x, 0, 2) plt.plot(x, y) plt.show()
The output of the above code will be:
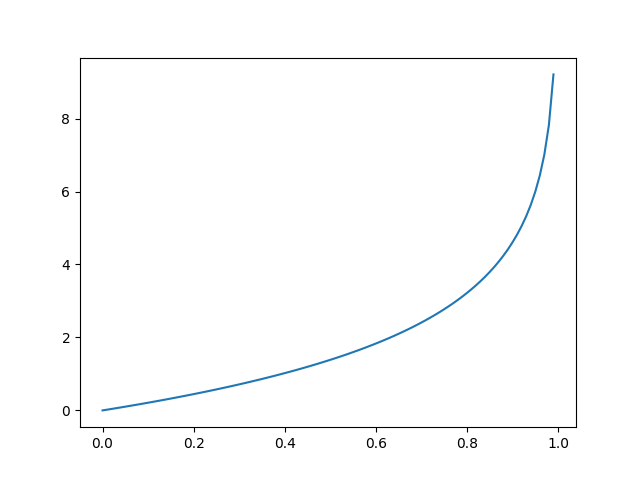
expon.rvs()
The expon.ppf() function generates an array containing specified number of random numbers of the given exponential distribution. In the example below, a histogram is plotted to visualize the result.
from scipy.stats import expon import matplotlib.pyplot as plt import numpy as np #fixing the seed for reproducibility #of the result np.random.seed(10) #creating a vector containing 10000 #exponentially distributed random numbers y = expon.rvs(0, 1, 10000) #creating bin bin = np.arange(-1,10,0.1) plt.hist(y, bins=bin, edgecolor='blue') plt.show()
The output of the above code will be:
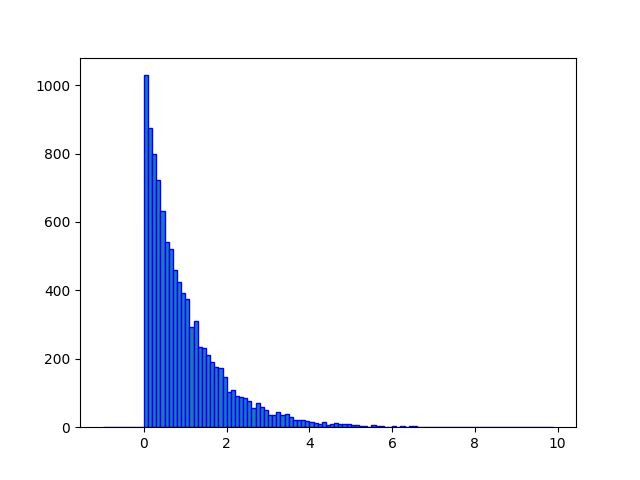