Python else Keyword
The Python else keyword is used to create conditional statements which executes when if and elif conditions gives false result.
Syntax
if condition: statements else: statements
Flow Diagram:
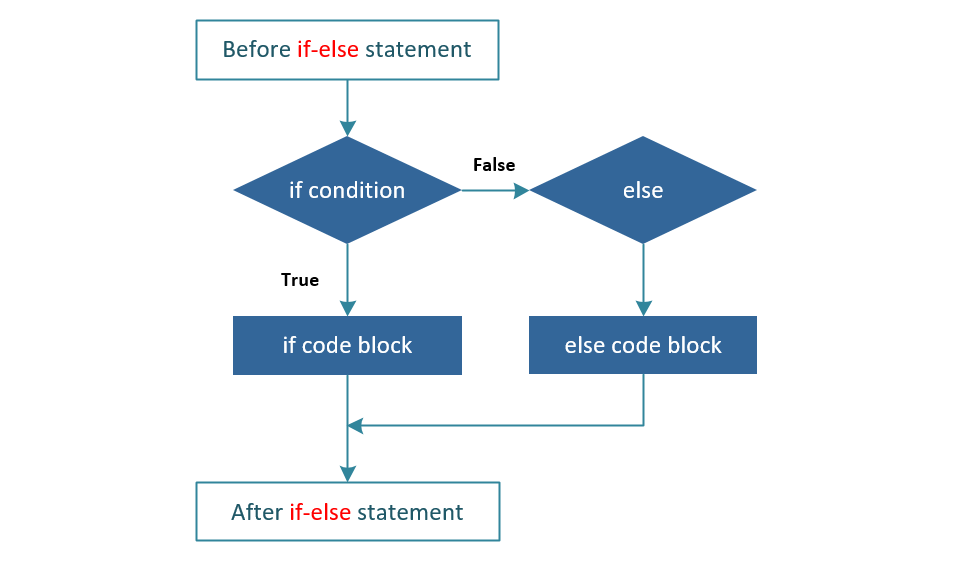
Example:
In the example below, else statement is used to print a message if the variable i is not divisible by 3.
i = 16 if i % 3 == 0: print(i," is divisible by 3.") else: print(i," is not divisible by 3.")
The output of the above code will be:
16 is not divisible by 3.
For adding more conditions, elif statement in used. The program first checks if condition. When found false, it checks elif conditions. If all elif conditions are found false, then else code block is executed.
Syntax
if condition: statements elif condition: statements ... ... ... else: statements
Flow Diagram:
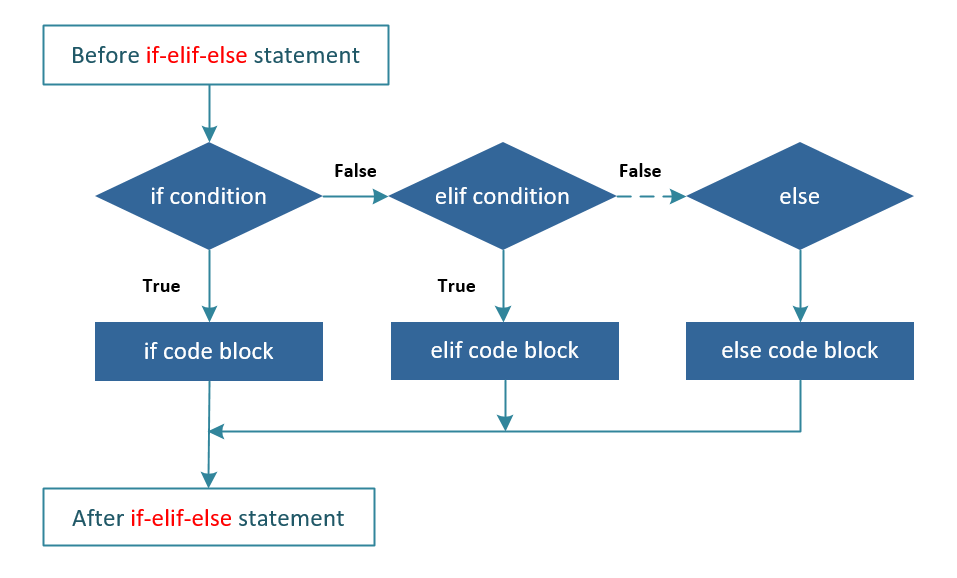
In the example below, elif statement is used to add more conditions between if statement and else statement.
i = 16 if i > 25: print(i," is greater than 25.") elif i <=25 and i >=10: print(i," lies between 10 and 25.") else: print(i," is less than 10.")
The output of the above code will be:
16 lies between 10 and 25.
❮ Python Keywords