R - For Loop
The For Loop
The for loop in R is used to iterate over a given sequence and executes a set of statements for each element in the sequence. A sequence can be any data structure like list, array, vector, and matrix etc.
Syntax
for iterating_var in sequence { statements }
Flow Diagram:
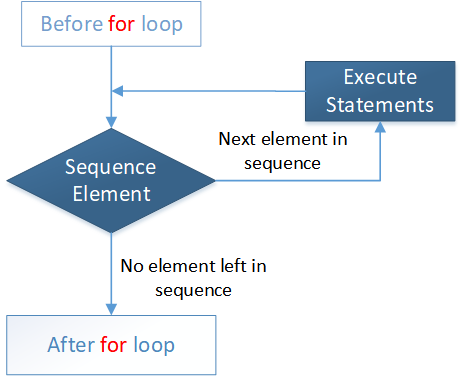
For loop over list
In the example below, for loop is used to print all elements of a given list.
weekday <- list("MON", "TUE", "WED", "THU", "FRI") #print all elements of weekday for (day in weekday) { print(day) }
The output of the above code will be:
[1] "MON" [1] "TUE" [1] "WED" [1] "THU" [1] "FRI"
For loop over vector
Similarly, it is used to print all elements of a vector.
number <- c(1:6) #print all elements of number for (i in number) { print(i) }
The output of the above code will be:
[1] 1 [1] 2 [1] 3 [1] 4 [1] 5 [1] 6
next statement
By using next statement, the program skips the loop whenever condition is met, and brings the program to the start of loop. Please consider the example below.
number <- c(1:10) #skips the loop for odd value of i for (i in number) { if(i %% 2 == 1) next print(i) }
The output of the above code will be:
[1] 2 [1] 4 [1] 6 [1] 8 [1] 10
break statement
By using break statement, the program get out of the for loop when condition is met. Please consider the example below.
number <- c(1:10) #get out of the loop when i == 5 for (i in number) { if(i == 5) break print(i) }
The output of the above code will be:
[1] 1 [1] 2 [1] 3 [1] 4