Matplotlib - PyLab
PyLab is a procedural interface to the Matplotlib and provides a Matlab like experience for the user. Matplotlib is the whole package, matplotlib.pyplot is a module in Matplotlib, and PyLab is a module that gets installed alongside Matplotlib.
PyLab imports portions of Matplotlib and NumPy. Many examples on the web use it as a simpler Matlab like experience, but it is not recommended anymore as it doesn't nurture understanding of Python itself, thus leaving the user in a limited environment.
Example: simple plot
Consider the example below where plot() function is used to plot y = sin(x). After that, the axes functions are used to format the axes. At last, show() function is used to display the figure.
from pylab import * #creating an array of values between #0 to 10 with a difference of 0.1 x = arange(0, 10, 0.1) y = sin(x) #plotting the curve plot(x, y) #formatting axes xlabel("x") ylabel("y = sin(x)") title("Sine wave") #displaying the figure show()
The output of the above code will be:
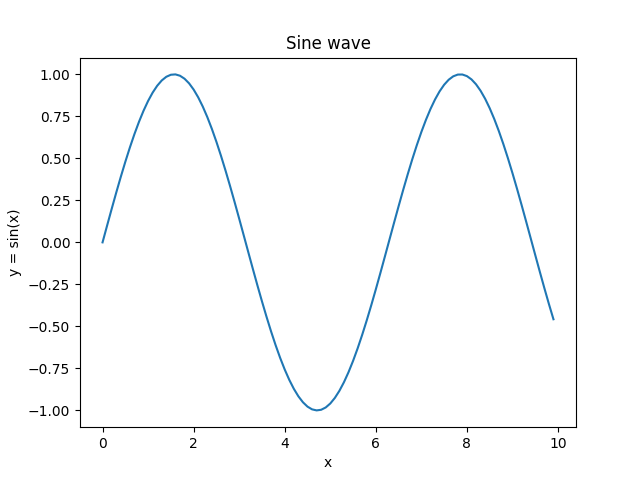
Example: adding legend
The example below shows how to add two plots in a single figure.
from pylab import * #creating an array of values between #0 to 10 with a difference of 0.1 x = arange(0, 10, 0.1) y1 = sin(x) y2 = cos(x) #plotting curves plot(x, y1) plot(x, y2) #formatting axes xlabel("x") ylabel("y") title("Sine vs Cosine") #adding legend legend(['sin(x)', 'cos(x)']) #displaying the figure show()
The output of the above code will be:
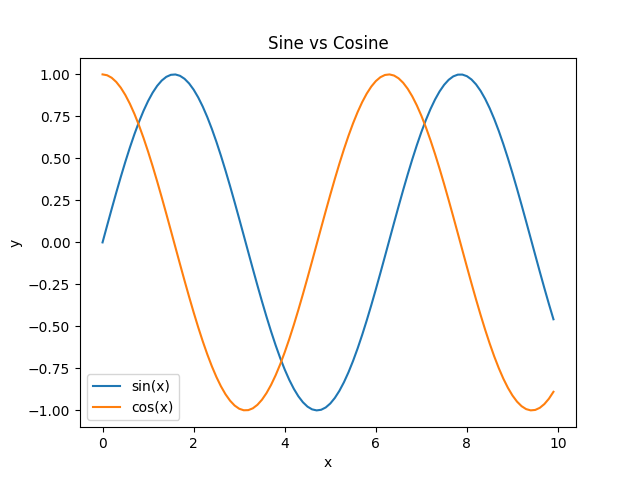
Format String
A format string consists of a part for color, marker and line:
fmt = '[marker][line][color]'
Each of them is optional. If not provided, the value from the style cycle is used. Other combinations such as [color][marker][line] are also supported, but note that their parsing may be ambiguous.
A format string can be added to a plot to add more styles in it.
Markers
Character | Description |
---|---|
'.' | point marker |
',' | pixel marker |
'o' | circle marker |
'v' | triangle_down marker |
'^' | triangle_up marker |
'<' | triangle_left marker |
'>' | triangle_right marker |
'1' | tri_down marker |
'2' | tri_up marker |
'3' | tri_left marker |
'4' | tri_right marker |
'8' | octagon marker |
's' | square marker |
'p' | pentagon marker |
'P' | plus (filled) marker |
'*' | star marker |
'h' | hexagon1 marker |
'H' | hexagon2 marker |
'+' | plus marker |
'x' | x marker |
'X' | x (filled) marker |
'D' | diamond marker |
'd' | thin_diamond marker |
'|' | vline marker |
'_' | hline marker |
Line styles
Character | Description |
---|---|
'-' | solid line style |
'--' | dashed line style |
'-.' | dash-dot line style |
':' | dotted line style |
Colors
Character | Description |
---|---|
'b' | blue |
'g' | green |
'r' | red |
'c' | cyan |
'm' | magenta |
'y' | yellow |
'k' | black |
'w' | white |
Example: using format string
In the example below, format string is used to add more styles in the plot.
from pylab import * #creating an array of values between #0 to 10 with a difference of 0.5 x = arange(0, 10, 0.5) y1 = sin(x) y2 = cos(x) #plotting curves plot(x, y1, 'o-r') plot(x, y2, 'v--b') #formatting axes xlabel("x") ylabel("y") title("Sine vs Cosine") #adding legend legend(['sin(x)', 'cos(x)']) #displaying the figure show()
The output of the above code will be:
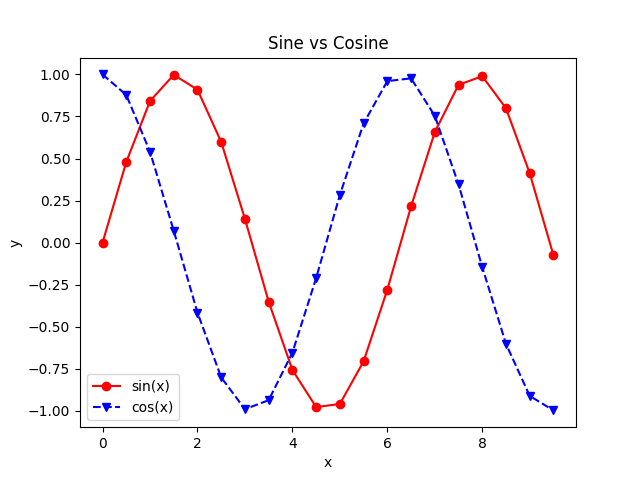