C# - For Loop
The for loop executes a set of statements in each iteration. Whenever, number of iteration is known, for loop is preferred over while loop.
Syntax
for(initialization(s); condition(s); counter_update(s);){ statements; }
- Initialization(s): Variable(s) is initialized in this section and executed for one time only.
- Condition(s): Condition(s) is defined in this section and executed at the start of loop everytime.
- Counter Update(s): Loop counter(s) is updated in this section and executed at the end of loop everytime.
Flow Diagram:
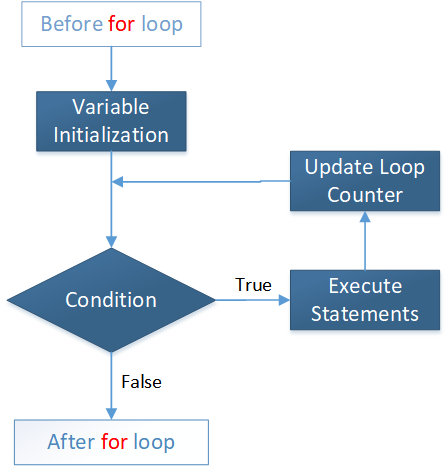
Example:
Multiple initializations, condition checks and loop counter updates can be performed in a single for loop. In the example below, two variable called i and j are initialized, multiple conditions are checked and multiple variables are updated in a single for loop.
using System; class MyProgram { static void Main(string[] args) { for (int i = 1; i <= 5; i++){ Console.WriteLine(i); } } }
The output of the above code will be:
1 2 3 4 5
Example
Multiple initializations, condition checks and loop counter updates can be performed in a single for loop. See the example below:
using System; class MyProgram { static void Main(string[] args) { for (int i = 1, j = 100; i <= 5 || j <= 800; i++, j = j + 100){ Console.WriteLine("i=" + i + ", j=" + j); } } }
The output of the above code will be:
i=1, j=100 i=2, j=200 i=3, j=300 i=4, j=400 i=5, j=500 i=6, j=600 i=7, j=700 i=8, j=800