C# - Arrays
Arrays are used to store multiple values in a single variable. In C#, all elements of an array must be of same datatype.
Create an Array
It starts with defining the datatype of the array, name of the array followed by size of the array enclosed in square brackets, [ ].
Initialize an Array
An array can be initialized by separating it's elements by comma , and enclosing with curly brackets { }. Please note that declaration of an array does not allocate memory for the array. To allocate the memory for an array, it must be initialized. If the array is not initialized at the time of declaration it must be initialized with new keyword followed by datatype and size of array enclosed in square bracket [ ]. See the syntax below for various methods of declaration and initialization.
Syntax
//declaration and initialization of //an integer array separately int[] MyArray; MyArray = new int[5]; //size of array is 5 MyArray[0] = 10; //first element is 10. //declaration and initialization of an string //array in a single line string[] MyArray = {"MON", "TUE", "WED"}; //Another method of declaration and initialization //of an string array in a single line string[] MyArray = new string[5]; //Another method of declaration and initialization //of an string array in a single line string[] MyArray = new string[3]{"MON", "TUE", "WED"};
Access element of an Array
An element of an array can be accessed with it's index number. In C#, the index number of an array starts with 0 as shown in the figure below:
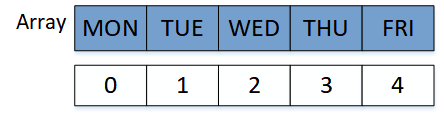
The example below describes how to access elements of an array using its index number.
using System; class MyProgram { static void Main(string[] args) { //creating an array with 5 elements string[] weekday = {"MON", "TUE", "WED", "THU", "FRI"}; //accessing the element with index number 4 Console.WriteLine(weekday[4]); } }
The output of the above code will be:
FRI
Modify elements of an Array
Any element of an Array can be changed using its index number and assigning a new value.
using System; class MyProgram { static void Main(string[] args) { string[] weekday = {"MON", "TUE", "WED", "THU", "FRI"}; weekday[0] = "MON_new"; weekday[3] = "THU_new"; Console.WriteLine(weekday[0]); Console.WriteLine(weekday[3]); } }
The output of the above code will be:
MON_new THU_new
Loop over an Array
With for loop or while loop, we can access each elements of an Array.
For Loop over an Array
In the example below, for loop is used to access all elements of the given array.
using System; class MyProgram { static void Main(string[] args) { string[] weekday = {"MON", "TUE", "WED", "THU", "FRI"}; for(int i = 0; i < 5; i++) { Console.WriteLine(weekday[i]); } } }
The output of the above code will be:
MON TUE WED THU FRI
While Loop over an Array
Similarly, while loop is also be used to access elements of the array.
using System; class MyProgram { static void Main(string[] args) { int[] numbers = {10, 20, 30, 40, 50}; int i = 0; while(i < 5) { Console.WriteLine(numbers[i]); i++; } } }
The output of the above code will be:
10 20 30 40 50
Multi-dimensional Array
Multi-dimensional array can be viewed as arrays of arrays. C# allows you to define array of any dimension.
Syntax
//2-dimension array int[,] MyArray; //3-dimension array int[,,] MyArray; //2-dimensional array with size (2,3) int[,] MyArray = new int[2,3]{{10,20,30},{40,50,60}};
using System; class MyProgram { static void Main(string[] args) { int[,] numbers = new int[2,3]{{10, 20, 30}, {40, 50, 60}}; for(int i = 0; i < 2; i++) { for(int j = 0; j < 3; j++) { Console.Write(numbers[i,j] + " "); } Console.WriteLine(); } } }
The output of the above code will be:
10 20 30 40 50 60