Swift - For-In Loop
The for-in loop in Swift is used to iterate over a given sequence and executes a set of statements for each element in the sequence. A sequence can be anything like array, string, range of numbers, tuple, set, and dictionary etc.
Flow Diagram:
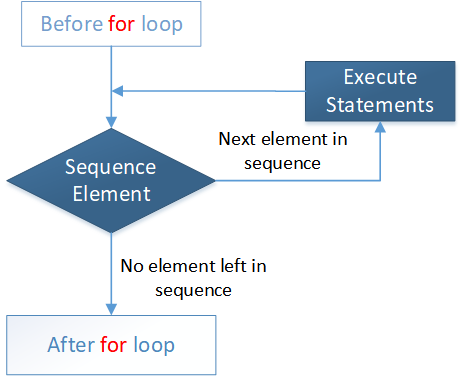
Iterating over a range of numbers
In the example below, the program uses for-in to iterate over range of numbers.
//iterating over a closed range to //print all elements in the range print("iterating over closed range") for i in (1...4) { print("i = \(i)") } //iterating over a half-open range to //print all elements in the range print("\niterating over half-open range") for j in (1..<4) { print("j = \(j)") }
The output of the above code will be:
iterating over closed range i = 1 i = 2 i = 3 i = 4 iterating over half-open range j = 1 j = 2 j = 3
Iterating using stride() function
The stride(from: to: by:) or stride(from: through: by:) function can be used to skip the unwanted marks.
//stride(from: to: by:) creates half-open range print("for-in loop using stride(from: to: by:)") for i in stride(from:1, to:9, by: 2) { print("i = \(i)") } //stride(from: through: by:) creates closed range print("\nfor-in loop using stride(from: through: by:)") for j in stride(from:1, through:9, by: 2) { print("j = \(j)") }
The output of the above code will be:
for-in loop using stride(from: to: by:) i = 1 i = 3 i = 5 i = 7 for-in loop using stride(from: through: by:) j = 1 j = 3 j = 5 j = 7 j = 9
Iterating over an Array
In the example below, the for-in is used to iterate over an array.
var MyArray:[Int] = [10, 20, 30, 40] //iterating over an array to print //all its elements print("MyArray contains:") for i in MyArray { print(i) }
The output of the above code will be:
MyArray contains: 10 20 30 40
Iterating over a Dictionary
In the example below, the for-in is used to iterate over a dictionary to access all key/value pairs.
var MyDict:[Int:String] = [1:"MON", 2:"TUE", 3:"WED", 4:"THU", 5:"FRI"] //iterating over a dictionary to print //all its elements print("MyDict contains:") for (key, value) in MyDict { print("\(key) = \(value)") }
The output of the above code will be:
MyDict contains: 5 = FRI 2 = TUE 3 = WED 4 = THU 1 = MON
When no iterating variable is required
Use _, if no iterating variable is required. Consider the example below:
var x = 2 var result = 1 var power = 10 //use _ when no iterating variable is required for _ in 1...power { result *= x } print("\(x) raised to the power of \(power) = \(result)")
The output of the above code will be:
2 raised to the power of 10 = 1024