Scala - For Loop
Scala for Loop
The for loop in Scala is used to iterate over a given sequence and executes a set of statements for each element in the sequence. A sequence can be any structure like ranges, strings, and collections like arrays and lists.
Syntax
for(variable <- sequence){ statements }
Flow Diagram:
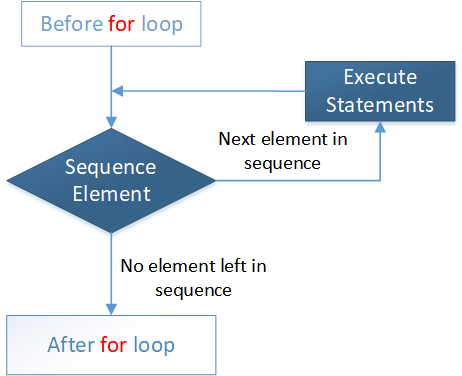
for loop over a range
In the example below, for loop is used over a given range to print all elements in it.
object MainObject { def main(args: Array[String]) { var i = 0 //for loop over a range for(i <- 1 to 5){ println("i = " + i) } } }
The output of the above code will be:
i = 1 i = 2 i = 3 i = 4 i = 5
In a for loop, multiple range can be separated using semicolon ;. When multiple ranges are used in a for loop, it will iterate through all the possible computations of the given ranges. Consider the example below:
object MainObject { def main(args: Array[String]) { var i = 0 var j = 0 //for loop over two ranges for(i <- 1 to 3; j <- 11 to 13){ println("i = " + i + ", j = " + j) } } }
The output of the above code will be:
i = 1, j = 11 i = 1, j = 12 i = 1, j = 13 i = 2, j = 11 i = 2, j = 12 i = 2, j = 13 i = 3, j = 11 i = 3, j = 12 i = 3, j = 13
for loop over a String
In the example below, for loop is used over a given string to print all characters of it, taking one character at a time in each iteration.
object MainObject { def main(args: Array[String]) { var c = "" //for loop over a string for(c <- "HELLO"){ println(c) } } }
The output of the above code will be:
H E L L O
for loop over an Array
In the example below, for loop is used over a given array to print all elements of it.
object MainObject { def main(args: Array[String]) { var i = 0 val MyArray = Array(10, 20, 30, 40, 50) //for loop over an array for(i <- MyArray){ println("i = " + i) } } }
The output of the above code will be:
i = 10 i = 20 i = 30 i = 40 i = 50
for loop over a List
Similarly, the for loop can be used over a list to print all elements of it. Consider the example below:
object MainObject { def main(args: Array[String]) { var i = 0 val MyList = List(100, 200, 300, 400, 500) //for loop over a list for(i <- MyList){ println("i = " + i) } } }
The output of the above code will be:
i = 100 i = 200 i = 300 i = 400 i = 500
for loop with Filters
Scala's for loop allows to filter out some elements by using if condition(s) with it. The syntax for using if condition(s) with a for loop is given below.
Syntax
for(variable <- sequence if condition 1 if condition 2 ...){ statements }
In the example below, a for loop is used over an array to print all its elements in the range [25, 50] and not a multiple of 10.
object MainObject { def main(args: Array[String]) { var i = 0 val MyArray = Array(15, 20, 25, 30, 35, 40, 45, 50, 55, 60) //for loop over an array with filters for(i <- MyArray if 25 to 50 contains i if i%10 != 0){ println("i = " + i) } } }
The output of the above code will be:
i = 25 i = 35 i = 45
for loop with yield
Scala allows to store the return values from a for loop. It can be achieved by using yield keyword. The following is the syntax:
Syntax
retval = for(variable <- sequence if condition 1 if condition 2 ...) yield variable //Note: {} can also be used instead of ()
In the example below, yield keyword is used to store result in a variable called retval.
object MainObject { def main(args: Array[String]) { var i = 0 val MyArray = Array(15, 20, 25, 30, 35, 40, 45, 50, 55, 60) //for loop over an array, yield keyword //is used to store i in retval var retval = for(i <- MyArray if 25 to 50 contains i if i%10 != 0) yield i //displaying the content of retval for(j <- retval) { println("j = " + j) } } }
The output of the above code will be:
j = 25 j = 35 j = 45
Consider one more example to learn more about using yield keyword with a for loop.
object MainObject { def main(args: Array[String]) { var i = 0 val MyArray = Array(15, 20, 25, 30, 35, 40, 45, 50, 55, 60) //for loop over an array, yield keyword //is used to store (i+ 100) in retval var retval = for(i <- MyArray if 25 to 50 contains i if i%10 != 0) yield (i + 100) //displaying the content of retval for(j <- retval) { println("j = " + j) } } }
The output of the above code will be:
j = 125 j = 135 j = 145