Scala - Math tan() Method
The Scala Math tan() method returns trigonometric tangent of an angle (angle should be in radians). In special cases it returns the following:
- If the argument is NaN or an infinity, then the result is NaN.
In the graph below, tan(x) vs x is plotted.
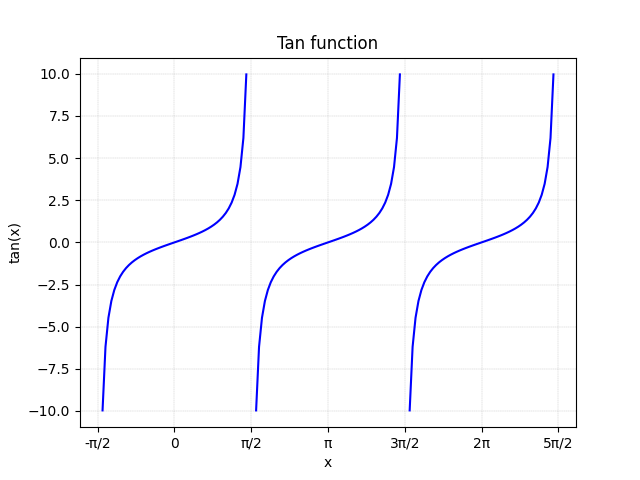
Syntax
def tan(x: Double): Double = scala.lang.Math.tan(x)
Parameters
x |
Specify the angle in radian. |
Return Value
Returns the trigonometric tangent of the argument.
Exception
NA.
Example:
In the example below, tan() method is used to find out the trigonometric tangent of the given angles.
import scala.math._ object MainObject { def main(args: Array[String]) { println(s"tan(Pi/6) = ${tan(Pi/6)}"); println(s"tan(Pi/4) = ${tan(Pi/4)}"); println(s"tan(Pi/3) = ${tan(Pi/3)}"); println(s"tan(Pi/2) = ${tan(Pi/2)}"); println(s"tan(Double.NaN) = ${tan(Double.NaN)}"); println(s"tan(2.0/0.0) = ${tan(2.0/0.0)}"); println("tan(Double.PositiveInfinity) = " + tan(Double.PositiveInfinity)); } }
The output of the above code will be:
tan(Pi/6) = 0.5773502691896257 tan(Pi/4) = 0.9999999999999999 tan(Pi/3) = 1.7320508075688767 tan(Pi/2) = 1.633123935319537E16 tan(Double.NaN) = NaN tan(2.0/0.0) = NaN tan(Double.PositiveInfinity) = NaN
❮ Scala - Math Methods