Java while Keyword
The Java while keyword is used to create a conditional statement loop called while loop which executes a set of statements repeatedly as long as a specified condition is true. The While loop can be viewed as a repeating if statement. There are two variants of while loop which is mentioned below:
- While loop: executes statements after checking the conditions.
- Do-While loop: executes statements before checking the conditions.
The While Loop
Syntax
while (condition) { statements; }
Flow Diagram:
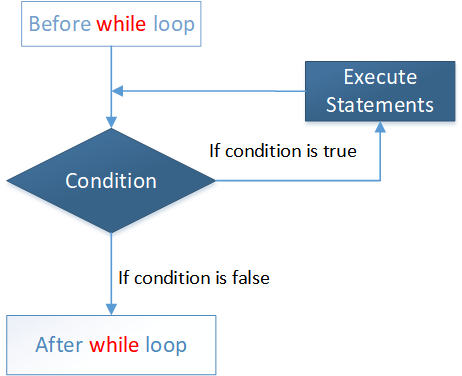
In below mentioned example, program uses while loop to sum all integers from 1 to 5.
public class MyClass { public static void main(String[] args) { int i = 0; int sum = 0; while (i <= 5) { sum = sum + i; i++; } System.out.println(sum); } }
The output of the above code will be:
15
The Do-While Loop
The Do-While loop in Java is a variant of while loop which execute statements before checking the conditions. Therefore the Do-While loop executes statements at least once.
Syntax
do { statements; } while (condition);
Flow Diagram:
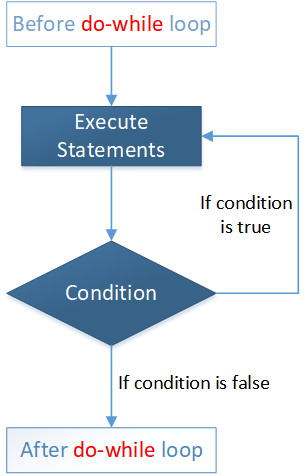
The above explained example is programmed using do-while loop and mentioned below:
public class MyClass { public static void main(String[] args) { int i = 0; int sum = 0; do{ sum = sum + i; i = i+1; } while (i <= 5); System.out.println(sum); } }
The output of the above code will be:
15
❮ Java Keywords