Java switch Keyword
The Java switch keyword is used to execute one of many code statements. It can be considered as group of If-else statements.
Syntax
switch (expression){ case 1: statement 1; break; case 2: statement 2; break; ... ... ... case N: statement N; break; default: default statement; }
The Switch expression is evaluated and matched with the cases. When Case matches, the following block of code is executed.
Flow Diagram:
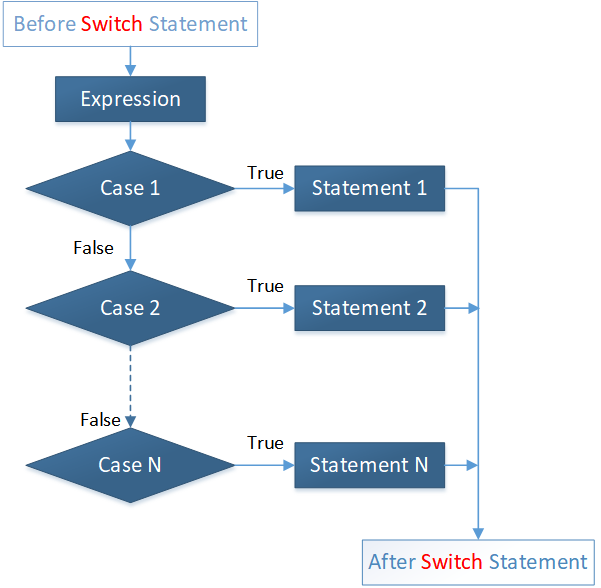
public class MyClass { public static void main(String[] args) { int i = 2; switch(i){ case 1: System.out.println("Red"); break; case 2: System.out.println("Blue"); break; case 3: System.out.println("Green"); break; } } }
The output of the above code will be:
Blue
Default case and break statement are optional here.
- Default Case: Default Statement is executed when there is no match between switch expression and test cases.
- Break Statement: Break statement is used to get out of the Switch statement after a match is found.
public class MyClass { public static void main(String[] args) { int i = 10; switch(i){ case 1: System.out.println("Red"); break; case 2: System.out.println("Blue"); break; case 3: System.out.println("Green"); break; default: System.out.println("There is no match in the switch statement."); } } }
The output of the above code will be:
There is no match in the switch statement.
❮ Java Keywords